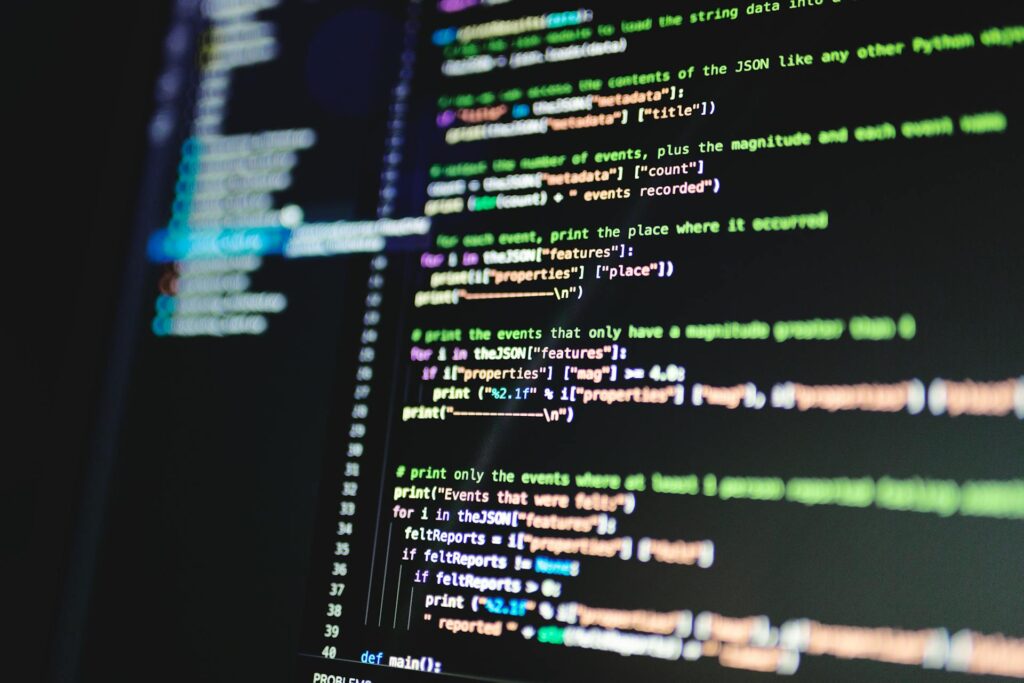
In software development, Angular unit testing plays a crucial role in ensuring code quality and reliability.
Angular applications are single-page applications that are built using the Angular framework. Most Angular applications have a component-based architecture and use HTML and TypeScript (a superset of JavaScript that adds static typing and other features).
As earlier stated, unit testing is a fundamental aspect of software development. It involves testing individual units of source code to determine whether they function as intended.
Isolating and testing each application unit makes it easy to detect bugs early in the development process. This facilitates refactoring, simplifies integrations, and improves code quality and maintenance.
Angular unit testing has specific characteristics that differentiate it from other frameworks besides the Angular framework. Some include using TestBed to write unit tests for Angular applications and testing specific features like data binding, component interaction, and routing.
RELATED POSTS
- 10 PROVEN PRACTICES FOR MOBILE APPLICATION DEVELOPMENT
- A STEP-BY-STEP GUIDE TO FULL-STACK WEB DEVELOPMENT MASTERY
- 10 EXPERT TIPS TO OPTIMIZE YOUR WEBSITE’S MOBILE RESPONSIVENESS
- 10 TOP PROGRAMMING LANGUAGES FOR WEB DEVELOPERS IN 2025
Understanding Angular Applications
The key to understanding Angular unit testing is understanding Angular applications and their uniqueness.
In simple terms, Angular applications are free, open-source, component-based, single-page web applications built using the Angular framework maintained by Google.
Here is an analogy to break this down for you.
Imagine building a house. Instead of laying every brick individually, you use pre-built modules, such as walls, windows, and doors, and assemble them to create the final house.
Angular is like that for websites. It’s a framework (a set of tools and rules) that lets you build websites using reusable “modules” called components.
So, instead of one big HTML file, an Angular website or application is made of smaller, manageable pieces (components). Each component has its HTML (what you see), CSS (how it looks), and JavaScript/TypeScript code (how it works).
The Angular framework can create scalable and interactive single-page web applications with dynamic content that changes based on the user’s interaction with the app.
Understanding Angular Unit Testing
Unit testing is especially crucial in Angular applications, which consist of various interconnected components. Unit testing allows you to verify the behavior of these components in isolation, ensuring the application’s overall stability and functionality.
The component-based architecture of Angular applications requires specific tools to test components in isolation properly.
This is where TestBed comes in.
TestBed is a unique and primary Angular API for writing unit tests. It configures and initializes the environment for unit testing by allowing you to:
- configure the testing environment for components
- compile components and their templates
- create component instances for testing
- provide mock dependencies or services
Setting Up Angular Unit Testing Environment
Setting up the proper environment is crucial before diving into the world of unit testing in Angular.
This setup’s cornerstone is installing two key tools: Jasmine and Karma.
Jasmine, a behavior-driven development framework, is the default testing framework for Angular unit testing that allows you to write clean, clear tests. It provides the syntax and structure for writing and organizing your unit tests.
On the other hand, Karma is a test runner designed to automate the testing process by executing tests in multiple browsers from the command line.
Once these tools are installed, the next step is creating and configuring your Angular project for unit testing. This step involves your Angular Command Line Interface (CLI) and TestBed.
You can create a new Angular project using the Angular CLI. However, to use it, you need to have knowledge of Javascript, HTML, and CSS.
The Angular CLI requires Node.js and npm (Node Package Manager) to be installed on your system. If you don’t have them, follow these steps:
- Install Node.js and npm (npm is included with Node.js.).
- Verify Node.js and npm Installation.
- Install the Angular CLI.
- Open your terminal or command prompt.
- Use the following command to install the Angular CLI:
npm install -g @Angular/cli
Get a detailed breakdown of installing your Angular CLI on the developer page.
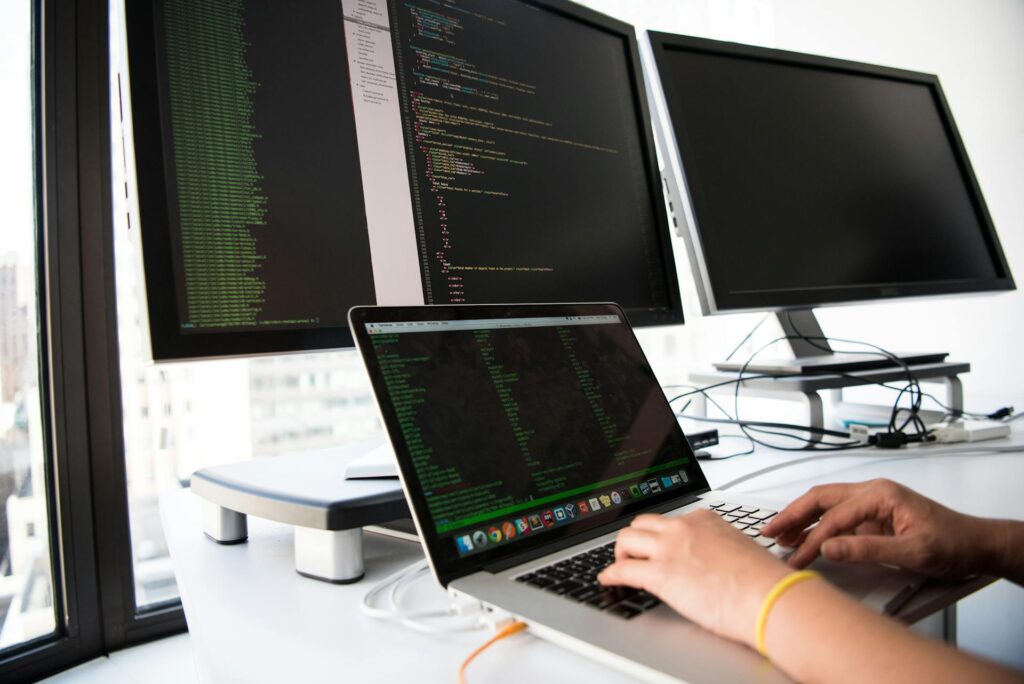
Angular Component Testing
Angular component testing involves validating the features of individual components, which make up the whole app’s creation. This process ensures that each component behaves as expected and leads to the overall stability of your Angular app.
A significant part of component testing involves interacting with the Document Object Model (DOM).
By simulating the resulting changes in DOM and user interactions, you can conclude that your parts respond accurately to user inputs.
Mocking Data and Faking Dependencies
Mocking should be considered in Angular unit testing. It involves creating mock objects that replicate the behavior of real objects, allowing you to isolate the unit of code under test.
This practice is critical in Angular, where components often depend on services or other components.
Creating fake dependencies, or “stubs,” is a common way to implement mocking in Angular testing. These fakes replace the actual dependencies, allowing you to control their behavior during testing. This control can be invaluable when you need to simulate specific scenarios or isolate your component from the rest of the application.
Jasmine, the testing framework commonly used with Angular, provides a feature called “spies” for this purpose. So, Jasmine spies can track calls to functions, fake return values, and even simulate errors, making them a powerful tool for your mocking needs.
Testing Asynchronous Code and Angular Observables
Asynchronous code presents a unique challenge for observables, allowing you to do unit tests. Due to its non-linear execution, traditional testing methods often need to catch up. However, Angular provides robust solutions to tackle this complexity, mainly when dealing with observables, a core feature of Angular’s asynchronous programming model.
Testing observables requires a different approach. Instead of testing the final state, you need to test the stream of values produced by the observable.
Angular’s testing utilities provide mechanisms to handle these asynchronous operations, allowing it to simulate and test various scenarios.
Measuring Code Coverage
Code coverage is a metric that quantifies the amount of your codebase tested by your suite of unit tests. It’s a valuable indicator of your tests’ comprehensiveness and the potential risks in your code.
In Angular, integrated with Karma, tools like Istanbul can measure code coverage. After your tests run, they generate a detailed coverage breakdown report.
Maintaining high code coverage is crucial. It reduces the risk of bugs, enhances code quality, and fosters confidence in your application’s reliability. However, coverage is not the sole indicator of test quality; your tests must also be meaningful and robust.
Follow these steps for your code coverage report:
- To generate a code coverage report, write the following command at the root of your Angular project:
ng test –no-watch –code-coverage
- To consistently create a code coverage report every time you run an Angular unit test, write the following command in your Angular CLI configuration file, angular.json:
“test”: {
“options”: {
“codeCoverage”: true
}
}
Writing Unit Tests
Writing unit tests in Angular involves examining the three fundamental elements of an Angular application, which include:
- Components
When testing components, focus on their behavior in isolation. It includes testing the component class, the template, and the interaction between the two.
Also, simulate user interactions, check the template’s rendering, and validate the component’s public API.
- Services
Testing services involves checking the methods and properties of the service.
- Pipes
Pipes transform input values into maintainable output values for display in a view. When testing pipes, provide input values and assert that the output is what we expect. It ensures that the service behaves as expected when its methods are called and maintains its state correctly.
Here’s an example of an automatically generated standard Angular unit test (TypeScript) for MyComponent.
import { ComponentFixture, TestBed } from ‘@Angular/core/testing’;
import { MyComponent } from ‘./my.component’;
describe(‘MyComponent’, () => {
let component: MyComponent;
let fixture: ComponentFixture<MyComponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [ MyComponent ]
})
.compileComponents();
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it(‘should create’, () => {
expect(component).toBeTruthy();
});
it(‘should have a title of “Hello World”‘, () => {
component.title = “Hello World”;
fixture.detectChanges();
const titleElement = fixture.nativeElement.querySelector(‘h1’);
expect(titleElement.textContent).toContain(‘Hello World’);
});
});
This sample test checks two things:
- that the component can be created
- that the component’s title property is correctly displayed in the template.
Running Unit Tests
Once your unit tests are written, it’s time to run them. Angular is typically done from the command line using the Angular CLI.
Note: Projects created using the Angular CLI are automatically ready for testing using a simple ng test command. This command initiates the Karma test runner, which executes your tests and reports the results in the console.
02 11 2022 09:08:28.605:INFO [karma-server]: Karma v6.4.1 server started at http://localhost:9876/
02 11 2022 09:08:28.607:INFO [launcher]: Launching browsers Chrome with concurrency unlimited
02 11 2022 09:08:28.620:INFO [launcher]: Starting browser Chrome
02 11 2022 09:08:31.312:INFO [Chrome]: Connected on socket -LaEYvD2R7MdcS0-AAAB with id 31534482
Chrome: Executed 3 of 3 SUCCESS (0.193 secs / 0.172 secs)
TOTAL: 3 SUCCESS
The last line of the log shows that all three tests run by Karma were successful.
But running tests is just part of the story.
In a modern development workflow, Angular unit tests are often run automatically in a continuous integration (CI) environment.
Continuous Integration
Continuous integration automates your Angular unit testing projects. This is often done to prevent the developer from forgetting to run frequent tests.
To do this, you link your unit testing to be triggered by specific events such as code commits, pull requests, or scheduled builds.
Use the following command to test your Angular CLI application in continuous integration (CI):
ng test –no-watch –no-progress –browsers=ChromeHeadless
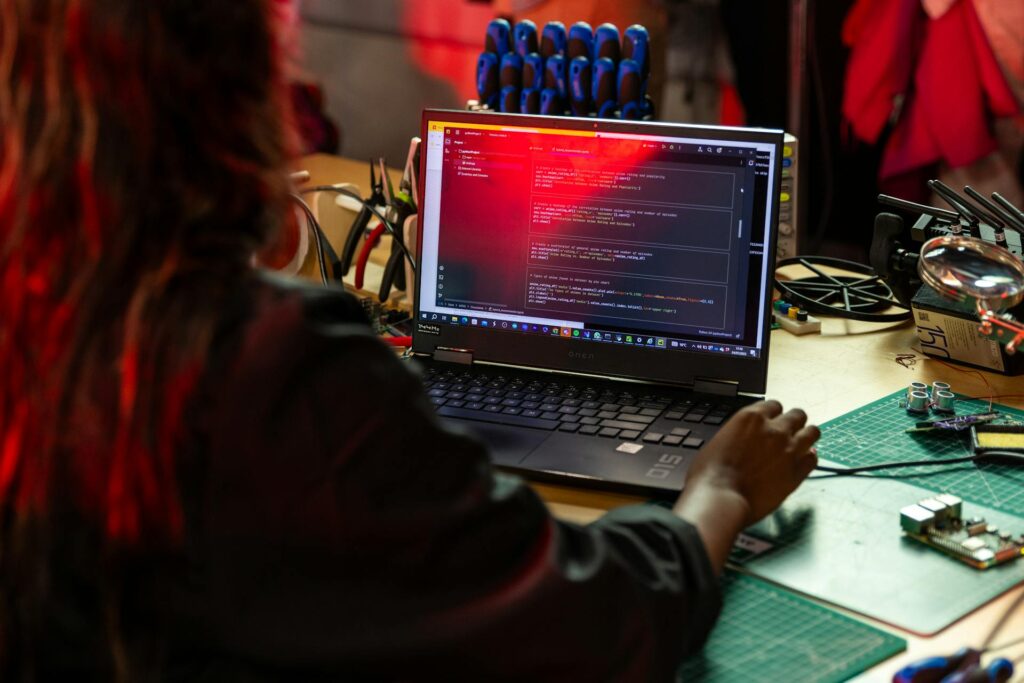
Conclusion
Effective Angular unit testing is both an art and a science. It requires a keen understanding of your application, a strategic approach, and a commitment to quality.
Therefore, you should consult a proficient company that can help you build robust applications and unit-test your Angular apps.
Avoid pitfalls such as neglecting edge cases, over-relying on positive tests, and testing implementation details. Instead, focus on testing behavior, covering all possible scenarios, and maintaining high code coverage.
If you want to conduct Angular unit testing for your website or application, email us at [email protected]